Core Data Management Guide for iOS App Development
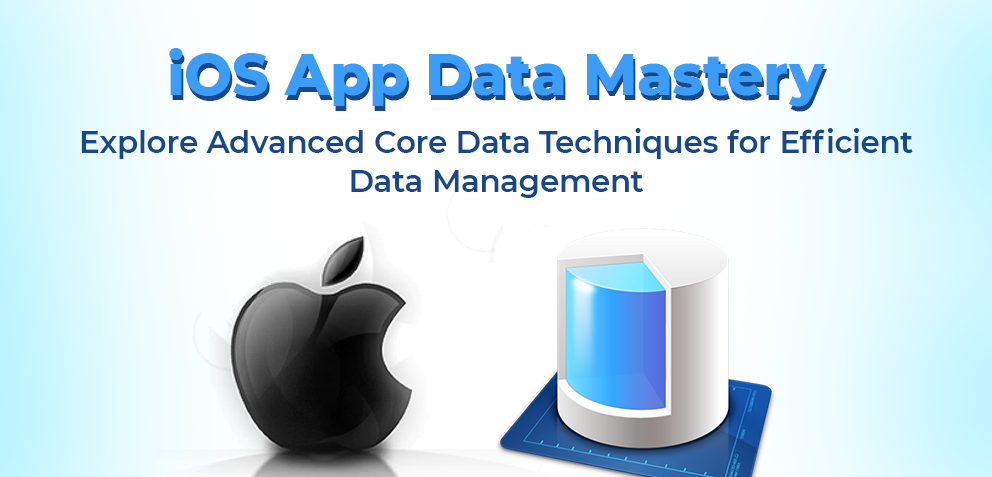
Tags
Category
- Adtech
- Android App Development
- API
- App Store
- Artificial Intelligence
- Blockchain Development
- Chatbot Development
- CMS Development
- Cybersecurity
- Data Security
- Digital Marketing
- Ecommerce Development
- Fintech
- Flutter app development
- Full Stack Development
- Hybrid App Development
- iOS App Development
- IT Project Management
- JavaScript development
- Laravel Development
- Magento Development
- MEAN Stack Developer
- Mobile App
- Mobile App Development
- Progressive Web Application
- python development
- QA and testing
- React Native
- SaaS
- SEO
- Shopify Development
- Software Development
- Software Outsourcing
- Staff Augmentation
- UI/UX Development
- Web analytics tools
- Wordpress Development
Mastering advanced Core Data techniques is imperative for optimal data management in iOS app development. This authoritative exploration delves into nuanced strategies, offering developers a profound understanding of efficient Core Data utilization.
From advanced querying to seamless synchronization, this discourse navigates the intricacies, empowering practitioners to elevate their proficiency in iOS app data handling.
Embark on a journey of excellence as we unravel the sophisticated layers of Core Data, providing indispensable insights for crafting high-performance, robust applications.
Understanding Memory Management in iOS App Development
Techniques to Optimize Data Handling in iOS App Development
- Core Data Review
- Advanced Fetching Techniques
- Concurrency in Core Data
- Performance Optimization
- Data Migration
- Batch Processing
- Faulting and Relationship Optimization
- Versioning and Lightweight Migration
- UI and Core Data Integration
- Error Handling and Debugging
- Testing Core Data
Best Practices and Design Patterns to Follow for Core Data Management in iOS App Development
Understanding Memory Management in iOS App Development
The first things first—Why do we need a memory management system?
Well, memory management in iOS is crucial for optimal app performance. Apple’s Automatic Reference Counting (ARC) is the primary mechanism, automatically managing memory by tracking object references. When an object’s reference count drops to zero, the associated memory is deallocated. Developers should adhere to ARC guidelines, understanding strong and weak references to prevent retain cycles.
Swift enhances memory safety with optionals and value types, minimizing memory-related issues. Efficient memory usage is imperative to avoid crashes and enhance user experience. Regularly monitoring memory usage using Instruments, addressing retain cycles, and optimizing code contribute to a robust memory management strategy in iOS development services.
Techniques to Optimize Data Handling in iOS App Development
Core Data Review
Core Data, a robust framework in iOS development, empowers efficient data management. At its core are: entities—representing objects—and attributes—defining their properties. Relationships establish connections between entities, fostering data coherence.
Core Data’s prowess lies in its ability to persistently store this information, ensuring seamless retrieval and modification. Mastering entities, attributes, and relationships is pivotal for crafting scalable and performant iOS apps.
This foundational understanding positions iPhone app developers to harness Core Data’s capabilities effectively, enhancing the overall user experience.
Advanced Fetching Techniques
Leverage fetch requests with sorting and filtering to tailor data responses to specific criteria, enhancing user experience. Optimize performance by fetching data in the background, ensuring a seamless and responsive app.
This approach minimizes UI lag and enhances overall efficiency. Always consider the balance between fetching relevant data and minimizing resource utilization to achieve an optimal user experience.
RELATED READ: UX Mastery: A Blueprint for Mobile App Development Success
Concurrency in Core Data
It involves managing simultaneous data access to ensure data consistency. Using multiple contexts, such as parent-child contexts, can optimize data handling in iOS apps. A parent context manages the persistent store, while child contexts perform operations and feed changes back to the parent.
This allows for efficient data processing without blocking the main thread. Changes made in child contexts are isolated until they are saved to the parent, minimizing conflicts.
Additionally, merging changes between contexts is handled seamlessly. This approach improves performance by distributing tasks across multiple threads, enhancing responsiveness and app experience.
Performance Optimization
It can be achieved through strategic indexing, especially when dealing with large datasets. Indexing accelerates data retrieval, enhancing fetch performance by allowing the database to locate specific records efficiently. Carefully selecting and creating indexes based on frequently queried attributes can significantly improve overall response times.
Efficient handling of large datasets involves employing techniques like pagination and asynchronous loading to prevent excessive memory usage. Fetching only required attributes further optimizes data handling by minimizing unnecessary data transfer, reducing network latency, and enhancing app responsiveness.
Utilizing Core Data efficiently, implementing background fetching, and employing multithreading or asynchronous operations contribute to a smoother user experience, ensuring the iOS app performs optimally even when dealing with substantial amounts of data.
Data Migration
Data migration strategies play a pivotal role in optimizing data handling within iOS apps. Lightweight approaches, such as incremental updates, prioritize efficiency by transferring only essential data. This minimizes disruption and ensures a seamless user experience. On the other hand, heavy migration approaches involve comprehensive overhauls, often suitable for substantial data model changes.
While they might incur more upfront effort, they offer long-term benefits in terms of improved data structure and performance. Careful consideration of the app’s complexity and user impact is crucial when choosing between these strategies.
Striking a balance between lightweight and heavy migration is essential for achieving optimal data handling and a smooth transition in the iOS app ecosystem.
Batch Processing
Batch processing in iOS app development refers to the efficient execution of a set of operations as a group, enhancing performance and minimizing resource consumption.
It involves grouping similar tasks, such as data updates, into a single batch to reduce overhead. Background contexts play a crucial role in optimizing data handling during batch operations. By employing background contexts, these operations can be performed asynchronously, preventing UI freezes and ensuring a seamless user experience. This approach maximizes efficiency by allowing the app to continue its essential functions while handling resource-intensive tasks in the background.
In summary, leveraging batch processing and background contexts is paramount for achieving optimal data management and responsiveness in iOS app development.
RELATED READ: Pro Tips for Optimal Performance in iOS App Development
Faulting and Relationship Optimization
In the realm of iOS app development, meticulous faulting comprehension is pivotal for superior memory management. Understanding faulting, such as lazy loading or fault-on-demand strategies, allows efficient resource utilization by loading data only when required. This minimizes memory footprint, enhancing overall app responsiveness.
Simultaneously, optimizing relationships is imperative for peak performance. Fine-tuning associations between data entities, employing appropriate Core Data relationships, and implementing fetching strategies enhance data handling efficiency.
Leveraging faulting and relationship optimization synergistically ensures optimal memory management and responsiveness in iOS applications.
Versioning and Lightweight Migration
This is pivotal for seamless data model evolution in iOS app development. When refining data models, adhere to Core Data best practices for versioning, ensuring compatibility across updates.
Employ lightweight migration techniques to smoothly transition between model versions, minimizing disruptions and preventing data loss. Leverage the Core Data model editor to make incremental changes, utilizing features like entity renaming and attribute restructuring.
Employ mapping models judiciously to guide the migration process intelligently. Rigorously test migrations to guarantee data integrity. By implementing strategic versioning and lightweight migration, your iOS app can efficiently adapt to evolving data structures, without risking valuable data.
UI and Core Data Integration
Integrating `NSFetchedResultsController` in your iOS app ensures efficient synchronization between Core Data and the UI. This powerful class monitors changes in the underlying data store, triggering dynamic updates to your UI seamlessly. By leveraging its delegate methods, you can handle insertions, deletions, and modifications with precision, minimizing performance overhead.
This approach optimizes data handling by avoiding manual UI updates and simplifying the management of fetched results. The controller efficiently tracks changes in the persistent store, delivering real-time updates to your interface without unnecessary reloading.
Utilizing `NSFetchedResultsController` is a cornerstone for maintaining a responsive and well-organized iOS app, aligning with best practices for effective Core Data integration.
Error Handling and Debugging
Common errors include data model mismatches, persistent store issues, and concurrency conflicts. To handle these, employ thorough validation before saving data, utilize try-catch blocks, and leverage Core Data’s error-handling mechanisms.
Debugging techniques involve utilizing breakpoints, examining fetched results, and utilizing the Core Data instrument in Xcode. Analyze SQL debug output for underlying store interactions, inspect managed object contexts, and validate relationships and fetch predicates. Optimize data handling by employing background contexts for heavy operations, batching fetch requests, and utilizing NSFetchedResultsController for efficient data presentation.
Overall, meticulous error handling, strategic debugging, and optimization techniques ensure a seamless Core Data integration, enhancing the reliability and performance of your iOS application.
RELATED READ: Mastering API Testing: A Comprehensive Guide to Success
Testing Core Data
For iOS unit testing strategies for Core Data, consider leveraging XCTest for robust testing. Employ mock objects to isolate Core Data dependencies, ensuring efficient and independent tests. Mocking Core Data facilitates controlled scenarios, allowing you to verify data handling without interacting with the actual persistent store.
Use dependency injection to substitute Core Data components with mocks during testing, promoting modular and scalable code. Employ XCTestExpectation for asynchronous testing, guaranteeing accurate evaluations.
Additionally, focus on testing CRUD operations, relationships, and error handling to ensure comprehensive coverage. These practices optimize data handling in your iOS app, enhancing reliability and maintainability while adhering to best practices in iOS development.
RELATED READ: Manual vs Automated Testing: What’s Best for You?
Best Practices and Design Patterns to Follow for Core Data Management in iOS App Development
When employing Core Data, adhere to best practices by designing a clear data model, utilizing lightweight migrations, and optimizing fetch requests for efficiency. Employ the MVC architecture, keeping business logic separate from the user interface. Implement the Singleton pattern for managing the Core Data stack, ensuring a single point of access.
Leverage the Repository pattern to encapsulate data access and modifications, promoting code maintainability. Employ the Observer pattern to enable communication between components without direct dependencies. Prioritize dependency injection for increased testability and flexibility.
Strive for modularity, encapsulating responsibilities in individual components. Regularly conduct code reviews to enforce these practices and ensure a scalable, maintainable codebase. This disciplined approach fosters a robust foundation for scalable applications while promoting code maintainability in the long run.
Conclusion
In summary, the adoption of advanced Core Data iOS Swift techniques is paramount for achieving optimal data management in iOS app development. Through meticulous implementation of features such as batch updates, prefetching, and efficient threading strategies, developers can significantly enhance the app’s performance and responsiveness.
This not only ensures a seamless user experience but also contributes to resource conservation and overall system efficiency.
Embracing these sophisticated Core Data practices reflects a commitment to excellence in app development, underscoring the importance of leveraging cutting-edge techniques for robust and efficient data handling in the dynamic landscape of iOS development. Moreover, hiring iOS developers is a great option for exploring data management techniques in iOS app development.
Read more on our Blog
Explore cutting-edge technologies with us. Dive into the latest tech stories, news, tips, and stay updated. Join our digital journey.